
Back to Blog
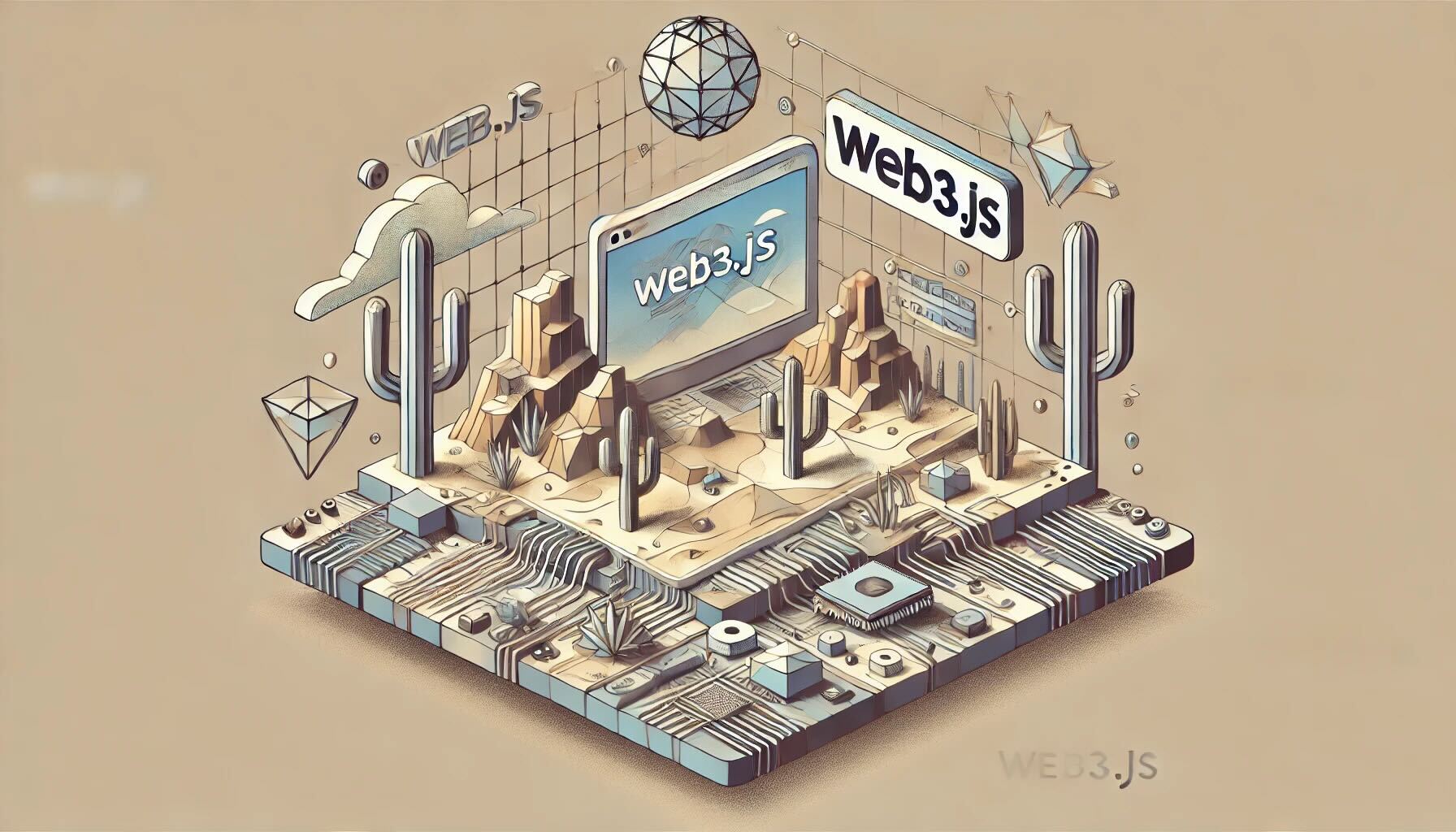
Now Available: Solana JavaScript SDK 2.0 Release Candidate
Written By
Anza Developers
August 1, 2024
If you build JavaScript applications on Solana, it’s likely that you’ve worked with @solana/web3.js
or a library powered by it. With 350K+ weekly downloads on npm, it’s the most-used library in the ecosystem for building program clients, web applications, backend services, mobile apps, and more.
In response to feedback from developers, we began a process of modernizing the library to prepare for the next generation of Solana applications. A Release Candidate of the new web3.js is now available.
This is an open invitation to test it out before the final release. Your feedback in these final moments will help us catch any bugs and rough edges that we missed during development.
What’s new?
Speed
Cryptographic operations like generating keypairs, signing transactions, and verifying messages are up to 10x faster in the new web3.js, thanks to its use of native Ed25519 cryptography APIs built into modern JavaScript runtimes like Node and Safari 17.
Efficiency
Applications that you develop with the new web3.js will be smaller, faster to load, and will have fewer dependencies. The new library itself is zero-dependency and can be tree-shaken by an optimizing compiler to reduce your bundle to only the parts of web3.js that you actually use.
Flexibility
Wherever your requirements deviate from the defaults we’ve set, you can compose our new networking, transaction confirmation, codec, and signing primitives with your own custom code. It’s newly possible to define RPC instances with custom methods, specialized network transports, custom transaction signers, and more, and we hope that you will open source them when you do.
Program Clients
New TypeScript clients for core on-chain programs are now available in the @solana-program org on GitHub. These clients are autogenerated from Kinobi IDLs meaning you can also use Kinobi to generate clients for your own programs. To get started, we recommend using the brand new pnpm create solana-program
script that spins up a new program with autogenerated clients out-of-the-box.
Quick Start
Fetch Data
Create a Transaction
Send And Confirm a Transaction
Try It
Install the Release Candidate using the rc
tag.
Try the runnable examples in the examples/ directory to get a feel for the API.
Use the example dApp at https://solana-labs.github.io/solana-web3.js/example/ – source available here – for an example of how to build transactions with the new web3.js for use with wallets.
Install TypeScript clients for on-chain programs like System and Token, then build a useful application or backend service.
Deep dive into the README at the root of the repository, and the individual READMEs in the subfolders of packages/ for much more detail.
Give Feedback
Before the general release of web3.js 2.0, shortly before Breakpoint 2024 in September, we want to collect as much feedback as possible from you. If you find a bug, are missing a feature, or would like an API modified, file a GitHub Issue.
Learn More
Watch the original announcement of the web3.js rewrite at Breakpoint 2023.